As development, staging and production environment are isolated from each other, so does the terraform code required to. In order to built a re-usable terraform code for both staging and production environment, without conducting copy and paste, one must follow the modules strategy. In this blog we will learn to Build AWS network via Terraform modules.
Terraform modules:
Think of them just like functions in a programming language, which we define once and then call them by passing parameters from anywhere in the code. Just like functions, put the infrastructure code inside a Terraform module and then reuse it in multiple places throughout the code.
Thus, both our staging and production environment (which are almost identical) can use the same modules without the requirement to copy and paste.
Let’s build a very basic and simple AWS network, comprising of a VPC, subnet and an EC2 instance using terraform modules.
Build AWS network via Terraform modules:
Step 1. Login to AWS console and putty into one of your instance (here we are using ubuntu instance).
Step 2. Install Terraform
Step 3. Install and configure AWS CLI.
Step 4. Create the following directory structure:
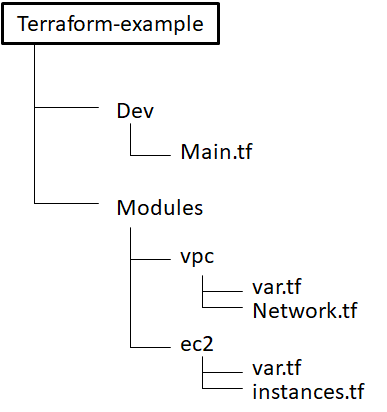
Step 5. Let’s start with writing code:
open the network.tf in an editor and write the following code:

//creating AWS network including VPC and subnet
resource "aws_vpc" "terraform_vpc" { cidr_block = var.vpc-fullcidr #### this 2 true values are for use the internal vpc dns resolution enable_dns_support = true enable_dns_hostnames = true tags = { Name = "terraform_vpc" } }
resource “aws_subnet” “subnet1” {
vpc_id =”${var.vpc_id}”
cidr_block = “${var.subnet_cidr}”
tags {
Name = “main”
}
output “vpc_id”{
value = “${aws_vpc.main.id}”
}
output “subnet_id” {
value = “${aws_subnet.subnet1.id}”
}
}
Step 6. Define variables:
In Step5 we have written the code to create vpc and subnet. However, rather hard coding, we have make use of variables. So lets define those variables inside modules –> vpc –> var.tf
//defining variables required by vpc module
variable “vpc_cidr” {
default = “10.0.0.0/16”
}
variable “tenancy” {
default = “dedicated”
}
variable “vpc_id” { }
variable “subnet_cidr” {
default = “10.0.1.0/24”
}
Step 7. Create ec2 module:
Edit instances.tf :

resource “aws_instance” “web-ec2” {
ami = “${var.ami_id}”
instance_type = “${var.instance_type}”
//put this ec2 instance inside a subnet which is inside a vpc
subnet_id = “${var.subnet_id}”
}
Step 8. Define variables for instances:
Edit var.tf inside modules –> ec2 –> var.tf
variable “ami_id” {}
variable “instance_type” {
default = “t2.micro”
}
variable “subnet_id” {}
variable “ec2_count” {
default = “1”
}
Step 9. Write code for dev environment
Edit main.tf
provider “aws” {
region = “${var.region}”
}
//Pass the parameters to the vpc module
module “my_vpc” {
source = “../modules/vpc”
vpc_cidr = “192.168.0.0/16”
tenancy = “default”
vpc_id = “${module.my_vpc.vpc_id}”
subnet_cidr = “192.168.1.0/24”
}
module “my_ec2” {
source = “../modules/ec2”
ec2_count = 1
ami_id = “ami-759bc50a”
instance_type =”t2.micro”
}
*Note:
vpc_id = “${module.my_vpc.vpc_id}”
Value of vpc_id has to be fetched from the module. To do so, define this value as output of the module, so that module can return the value of the output variable. And the above is the syntax to access it in the user/dev environment.
Step 10. edit var.tf of dev folder
variable “region” {
default =”us-east-1″
}
Step 11. Following commands are used to create the infrastructure via terraform:
terraform-example/dev$ terraform init
terraform-example/dev$ terraform apply
Check your AWS console, to see the successful creation of VPC, subnet and ec2 instance.